The LIKE operator is used in SQL to compare a string to a pattern. It is often used with the wildcard characters โ%โ and โ_โ. The โ%โ character matches any sequence of zero or more characters and the โ_โ character matches any single character. The LIKE operator is commonly used in conjunction with the SELECT statement to retrieve data that matches a certain pattern. In this article, we will explore the SQL LIKE operator with examples from the Northwind database in SQL Server.
The Northwind database is a sample database that is commonly used for SQL Server training and testing purposes. It contains tables for customers, orders, products, and other entities related to a fictional company that sells food products. We will be using the Customers and Orders tables in the Northwind database to illustrate the use of the LIKE operator.
Using the SQL LIKE Operator with the โ%โ Wildcard
Letโs start by using the โ%โ wildcard with the LIKE operator to retrieve customers whose company names contain the word โfoodโ. Hereโs the SQL query:
SELECT * FROM Customers
WHERE CompanyName LIKE '%food%';
This query will retrieve all customers whose CompanyName contains the word โfoodโ. The โ%โ wildcard matches any sequence of zero or more characters, so this query will match โFoodies Inc.โ, โFood for Thoughtโ, โGood Food Marketโ, and any other customer whose CompanyName contains the word โfoodโ.
Using the SQL LIKE Operator with the โ_โ Wildcard
Now letโs use the โ_โ wildcard with the LIKE operator to retrieve customers whose company names contain exactly four characters. Hereโs the SQL query:
SELECT * FROM Customers
WHERE CompanyName LIKE '________';
This query will retrieve all customers whose CompanyName contains exactly 8 characters. The โ_โ wildcard matches any single character, so this query will match any customer whose CompanyName is exactly 8characters long.
Here is the result of that query n SQL Server:

Using the SQL LIKE Operator with Multiple Wildcards
We can also use multiple wildcards in a single LIKE operator. For example, letโs retrieve all orders whose ShipCity starts with the letter โBโ and ends with the letter โoโ. Hereโs the SQL query:
SELECT * FROM Orders
WHERE ShipCity LIKE 'B%o';
This query will retrieve all orders whose ShipCity starts with โBโ and ends with โoโ. The โ%โ wildcard matches any sequence of zero or more characters, so this query will match โBostonโ, โBordeauxโ, โBoltonโ, and any other ShipCity that starts with โBโ and ends with โoโ.
Here are some more examples of how the SQL LIKE operator can be used to search for patterns in text data:
Retrieve all products whose product name starts with โChaiโ
SELECT * FROM Products
WHERE ProductName LIKE 'Chai%';
This query will retrieve all products whose ProductName starts with the word โChaiโ, such as โChaiโ, โChai Latteโ, โChai Teaโ, and so on.
Combining LIKE with the OR Operator
Retrieve all customers whose contact title is either โOwnerโ or โManagerโ
SELECT * FROM Customers
WHERE ContactTitle LIKE '%Owner%' OR ContactTitle LIKE '%Manager%';
This query will retrieve all customers whose ContactTitle contains either the word โOwnerโ or the word โManagerโ. Note that we use the โ%โ wildcard to match any sequence of zero or more characters before or after the search term.
Retrieve all orders whose ship country is either โUSAโ or โCanadaโ
SELECT * FROM Orders
WHERE ShipCountry LIKE 'U%S%A' OR ShipCountry LIKE 'Canada';
This query will retrieve all orders whose ShipCountry is either โUSAโ or โCanadaโ. Note that we use the โ%โ wildcard in this case to get all the cases where country is fpr example โU.S.Aโ.
Combining LIKE with the AND Operator
Retrieve all customers whose company name contains the word โfoodโ and whose city starts with the letter โSโ:
SELECT * FROM Customers
WHERE CompanyName LIKE '%food%' AND City LIKE 'S%';
This query will retrieve all customers whose CompanyName contains the word โfoodโ and whose City starts with the letter โSโ, such as โFoodies Inc.โ located in โSeattleโ, โGood Food Marketโ located in โSan Franciscoโ, and so on.
Retrieve all orders whose ship country is either โUSAโ or โCanadaโ and whose ship city contains the word โvilleโ:
SELECT * FROM Orders
WHERE ShipCountry LIKE 'USA' OR ShipCountry LIKE 'Canada'
AND ShipCity LIKE '%ville%';
This query will retrieve all orders whose ShipCountry is either โUSAโ or โCanadaโ and whose ShipCity contains the word โvilleโ, such as โMontrealโ in Canada and โLouisvilleโ in the USA.
Combining LIKE with Regular Expressions
Retrieve all products whose product name ends with โaโ, โbโ, โcโ, or โdโ
SELECT * FROM Products
WHERE ProductName LIKE '%[a-d]';
This query selects all rows from the โProductsโ table where the โProductNameโ column ends with any character that is either โaโ, โbโ, โcโ, or โdโ. The โ%โ wildcard is used to match any sequence of zero or more characters before the character set, and the character set itself matches any one of the specified letters at the end of the string.
So, this query will return all products whose names end with any one of the letters โaโ, โbโ, โcโ, or โdโ. For example, it would match โIkuraโ, โTunnbroidโ, โRogede Soidโ, โPavlovaโ, and many other products that end with these letters. It will not match any products whose names do not end with these letters.
Note that the letter range specified in the character set is case-sensitive, so it will only match uppercase or lowercase versions of the specified letters. To match both uppercase and lowercase versions of the letters, you could use a character set like this: โ%[a-dA-D]โ.
Here is the result of this query in SQL Server.
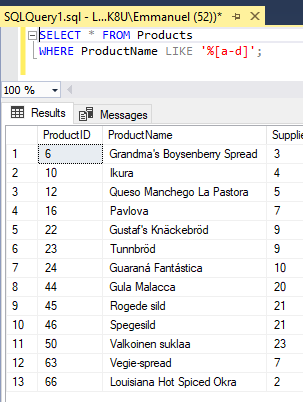
Using LIKE with Regular Expressions and a Subquery
Retrieve all orders for which contact name ends with โaโ, โbโ, โcโ, or โdโ
SELECT * FROM Orders
WHERE CustomerID IN (
SELECT CustomerID FROM Customers
WHERE ContactName LIKE '%[aeiou]%'
);
This query selects all rows from the โOrdersโ table where the โCustomerIDโ column contains any value that matches a value in the โCustomerIDโ column of the โCustomersโ table, and where the โContactNameโ column of the matching customer contains any string of characters that ends with any character that is either โaโ, โbโ, โcโ, or โdโ. The โ%โ wildcard is used to match any sequence of zero or more characters before the character set, and the character set itself matches any one of the specified letters at the end of the string.
So, this query will return all orders that were placed by customers whose ContactName ends with any one of the letters โaโ, โbโ, โcโ, or โdโ. The subquery first selects all customer IDs from the โCustomersโ table whose ContactName column ends with any one of these letters (returns 10 CustomerIDs), and then the main query selects all orders from the โOrdersโ table whose CustomerID matches one of the customer IDs returned by the subquery.
Note that the letter range specified in the character set is case-sensitive, so it will only match uppercase or lowercase versions of the specified letters. To match both uppercase and lowercase versions of the letters, you could use a character set like this: โ%[a-dA-D]โ.
Here is the result of this query in SQL Server:
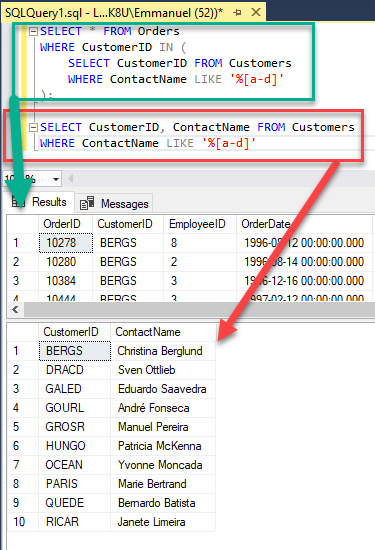
Conclusion
The LIKE operator is a powerful tool for searching for patterns in text data in SQL. It provides a flexible and intuitive way to filter records based on specific text patterns by using various wildcard characters such as โ%โ, โ_โ, and โ[]โ. It allows for searching for specific text patterns that match a certain format, or for finding records that contain specific characters or character ranges.
The LIKE operator is widely used in SQL queries and is supported by many database management systems. However, it can be relatively slow when used on large datasets, and using regular expressions in combination with LIKE may be a more efficient way to search for complex patterns.
Overall, the LIKE operator is an essential tool for searching text data in SQL, and it is important for developers and database administrators to have a good understanding of its syntax and functionality in order to efficiently query and manipulate data in their databases.
Leave a Reply